Ara
Enumerators
- koraylimancre
- 14 Oca 2022
- 1 dakikada okunur
Güncelleme tarihi: 15 Oca 2022
Enumeration is a method to define data types and assign them to values. By default first data type is assigned to 0, second data type is assigned to 1, third one is assigned to 2 and so on
#include <iostream>
enum State
{
Idle,
Walking,
Running,
Jumping
};
/*
enum State
{
Idle = 0,
Walking = 5,
Running = 10,
Jumping = 15
};
*/
int main()
{
State Playerstate = Idle;
switch (Playerstate)
{
case Idle:
// SomeFunc();
break;
case Walking:
// SomeFunc1();
break;
case Running:
// SomeFunc2();
break;
case Jumping:
// SomeFunc3();
break;
default:
break;
}
std::cout << Playerstate << std::endl;
Playerstate = Walking;
std::cout << Playerstate << std::endl;
Playerstate = Running;
std::cout << Playerstate << std::endl;
Playerstate = Jumping;
std::cout << Playerstate << std::endl;
}
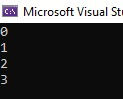
We also have enum class type which is called "strongly-typed" or "scoped". It is more secure to use and should be preferred over enum type in most cases. Since this type has no implicit conversion, you have cast it manually(explicit conversion) such as an int like this "int(data)".
#include <iostream>
/*
enum class State
{
Idle,
Walking,
Running,
Jumping
};
*/
enum class State
{
Idle = 0,
Walking = 5,
Running = 10,
Jumping = 15
};
int main()
{
State Playerstate = State::Idle;
switch (Playerstate)
{
case State::Idle:
// SomeFunc();
break;
case State::Walking:
// SomeFunc1();
break;
case State::Running:
// SomeFunc2();
break;
case State::Jumping:
// SomeFunc3();
break;
default:
break;
}
std::cout << int(Playerstate) << std::endl;
Playerstate = State::Walking;
std::cout << int(Playerstate) << std::endl;
Playerstate = State::Running;
std::cout << int(Playerstate) << std::endl;
Playerstate = State::Jumping;
std::cout << int(Playerstate) << std::endl;
}
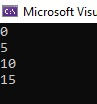
Comments